How to Create a Chatbot with ChatGPT
Learn how to create a chatbot with ChatGPT in this step-by-step guide. Build an engaging, intelligent chatbot using OpenAI's API and Python.
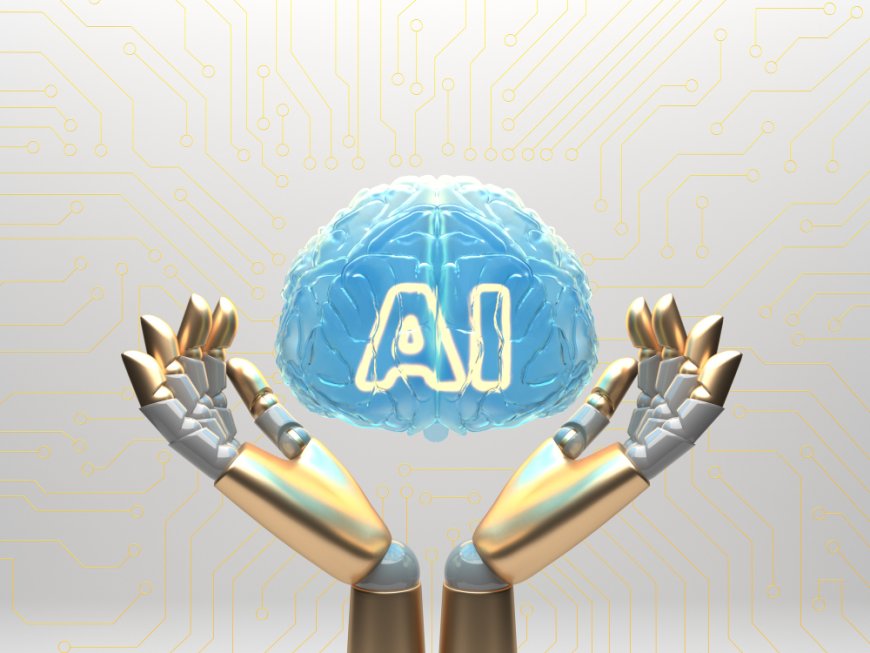
Building a chatbot powered by advanced AI is an exciting project, and I find ChatGPT to be a game-changer for creating intelligent conversational agents. We, as developers, can harness its capabilities to craft chatbots that engage users effectively. They, the users, expect natural, responsive interactions, making ChatGPT an ideal tool. This blog post outlines the steps to master how to create a chatbot with ChatGPT, covering setup, integration, customization, and deployment.
By following this approach, we ensure the chatbot is functional and user-friendly. Whether you’re a beginner or seasoned coder, I aim to make how to create a chatbot with ChatGPT accessible and practical.
Setting Up the Development Environment
To start learning how to create a chatbot with ChatGPT, we need a robust development environment. I recommend installing Python, as it’s the primary language for interacting with OpenAI’s API, which powers ChatGPT. They, the developers, often use IDEs like PyCharm or Visual Studio Code for efficient coding. Additionally, we’ll need an OpenAI account to access the API.
Key setup steps:
-
Install Python: Download Python 3.8 or later from python.org.
-
Choose an IDE: Use PyCharm for advanced features or VS Code for simplicity.
-
Set Up OpenAI Account: Sign up at openai.com and generate an API key.
-
Install Libraries: Use pip to install openai and requests for API calls.
Verify the setup by running python --version in the terminal. This ensures how to create a chatbot with ChatGPT begins smoothly. However, secure your API key to prevent unauthorized access.
Obtaining and Configuring the OpenAI API
The OpenAI API is central to how to create a chatbot with ChatGPT. I find it straightforward to configure once you have an API key. They, the OpenAI team, provide clear documentation for integrating ChatGPT’s capabilities. We need to set up the API client in Python to send and receive messages.
Example API configuration:
import openai
openai.api_key = "your-api-key-here"
Store the key in an environment variable for security. For specialized applications, like an adult AI chatbot, we must ensure compliance with OpenAI’s content policies, which prohibit explicit material. Specifically, testing the API with sample queries helps verify connectivity. Thus, mastering how to create a chatbot with ChatGPT hinges on proper API setup.
Designing the Chatbot’s Functionality
Defining the chatbot’s purpose is crucial for how to create a chatbot with ChatGPT. I suggest outlining its role—whether for customer support, education, or entertainment. They, the users, benefit from a clear scope, such as answering FAQs or roleplaying scenarios. We can design the chatbot to handle specific domains by crafting tailored prompts.
Key functionality considerations:
-
Conversation Scope: Define topics like tech support or general knowledge.
-
Tone and Style: Set a friendly, formal, or humorous tone via prompts.
-
Context Handling: Maintain conversation history for coherent responses.
-
Fallback Responses: Program defaults for out-of-scope queries.
For instance, when exploring how to create a chatbot with ChatGPT, we might design an NSFW AI chatbot with strict moderation to align with OpenAI’s guidelines. In comparison to generic chatbots, domain-specific ones require precise prompts. However, overly rigid prompts can limit flexibility, so balance is key.
Crafting Effective Prompts
Prompt engineering is at the core of how to create a chatbot with ChatGPT. I find that well-crafted prompts guide ChatGPT to produce relevant, accurate responses. They, the prompts, act as instructions, setting the chatbot’s behavior and context. We can use system messages to define the chatbot’s persona.
Example prompt:
messages = [
{"role": "system", "content": "You are a helpful tech support assistant."},
{"role": "user", "content": "My laptop won’t boot. Help!"}
]
response = openai.ChatCompletion.create(model="gpt-3.5-turbo", messages=messages)
This code sets a tech support persona. For niche applications, like an AI porn generator, prompts must avoid explicit content to comply with OpenAI’s policies. Specifically, iterative prompt testing improves response quality. Thus, how to create a chatbot with ChatGPT relies heavily on effective prompts.
Building the Conversation Logic
The conversation logic determines how to create a chatbot with ChatGPT that feels natural. I recommend maintaining a message history to ensure context-aware responses. They, the chatbots, need to track previous interactions to avoid repetitive or disjointed replies. We can implement this using a list to store user and assistant messages.
Example conversation loop:
history = []
while True:
user_input = input("You: ")
history.append({"role": "user", "content": user_input})
response = openai.ChatCompletion.create(model="gpt-3.5-turbo", messages=history)
reply = response.choices[0].message.content
history.append({"role": "assistant", "content": reply})
print("Bot:", reply)
This loop maintains context. For specialized chatbots, like an AI porn video generator, we must filter inputs to prevent policy violations. In the same way, limiting history size prevents token overload. Consequently, how to create a chatbot with ChatGPT requires robust logic to sustain engaging dialogues.
Creating a User Interface
A user-friendly interface enhances how to create a chatbot with ChatGPT. I suggest using Python frameworks like Flask for web-based interfaces or Tkinter for desktop GUIs. They, the users, interact through a text input field and chat display. For web deployment, Flask serves HTML templates with CSS styling.
Key UI components:
-
Input Field: Allows users to type messages.
-
Chat Display: Shows conversation history with timestamps.
-
Send Button: Triggers message processing.
-
Clear Chat Option: Resets the conversation for fresh starts.
For example, when learning how to create a chatbot with ChatGPT, a Flask-based UI can support real-time chats. However, web interfaces require hosting, unlike Tkinter’s local execution. In comparison to complex frameworks, Flask is lightweight, ideal for how to create a chatbot with ChatGPT.
Integrating Content Moderation
Content moderation is essential for how to create a chatbot with ChatGPT, especially for sensitive applications. I find OpenAI’s moderation API effective for flagging inappropriate inputs. They, the developers, must ensure compliance with usage policies to avoid account suspension. We can integrate moderation before processing user inputs.
Example moderation check:
moderation = openai.Moderation.create(input=user_input)
if moderation.results[0].flagged:
print("Input blocked due to policy violation.")
else:
# Process input
For chatbots resembling an adult AI chatbot, moderation prevents explicit content, ensuring safety. Specifically, logging flagged inputs helps refine prompts. Thus, how to create a chatbot with ChatGPT demands vigilant moderation to maintain trust.
Testing the Chatbot’s Performance
Testing ensures how to create a chatbot with ChatGPT results in a reliable product. I recommend evaluating response accuracy, context retention, and error handling. They, the testers, should simulate diverse user scenarios, including ambiguous or off-topic queries.
Testing checklist:
-
Accuracy: Verify responses align with the chatbot’s purpose.
-
Context: Check if conversation history is maintained correctly.
-
Edge Cases: Test long inputs or special characters.
-
Moderation: Ensure inappropriate inputs are flagged.
For instance, when mastering how to create a chatbot with ChatGPT, we test how it handles NSFW queries to confirm filter effectiveness. However, extensive testing can increase costs due to API usage. As a result, prioritize high-impact test cases for how to create a chatbot with ChatGPT.
Deploying the Chatbot
Deploying the chatbot is a critical step in how to create a chatbot with ChatGPT. I suggest using cloud platforms like Heroku, AWS, or Google Cloud for scalability. They, the platforms, support Python applications with easy setup. For web-based chatbots, Flask apps can be deployed as containers.
Deployment steps:
-
Package Code: Bundle the app using a requirements.txt file.
-
Configure Hosting: Set up Heroku or AWS with environment variables for the API key.
-
Test Deployment: Verify the chatbot responds correctly online.
-
Monitor Logs: Track errors and performance metrics.
For example, deploying an AI chatbot requires strict compliance with hosting platform policies. In comparison to local servers, cloud deployment ensures accessibility but incurs costs. Hence, how to create a chatbot with ChatGPT culminates in a globally available service.
Maintaining and Updating the Chatbot
Ongoing maintenance is vital for how to create a chatbot with ChatGPT. I find that monitoring user feedback and API updates improves performance. They, the developers, should refine prompts and logic based on real-world usage. We can also implement analytics to track engagement.
Maintenance tasks:
-
Feedback Review: Analyze user complaints or suggestions.
-
Prompt Updates: Adjust prompts for better accuracy.
-
API Versioning: Upgrade to newer ChatGPT models as released.
-
Security Checks: Ensure API keys and data remain secure.
For instance, maintaining an NSFW AI chatbot involves frequent moderation audits. Similarly, analytics help identify popular features. Thus, how to create a chatbot with ChatGPT requires continuous care to stay relevant.
Conclusion
Mastering how to create a chatbot with ChatGPT opens doors to building intelligent, engaging conversational agents. I believe that by setting up the environment, crafting prompts, and deploying thoughtfully, we can create chatbots that delight users.
They, the users, benefit from seamless interactions tailored to their needs. From designing functionality to maintaining the system, each step in how to create a chatbot with ChatGPT builds a robust product. We, as developers, can take pride in delivering innovative solutions with ChatGPT’s power.