How to Build Your First Project in ASP.NET: A Complete Guide for Beginner
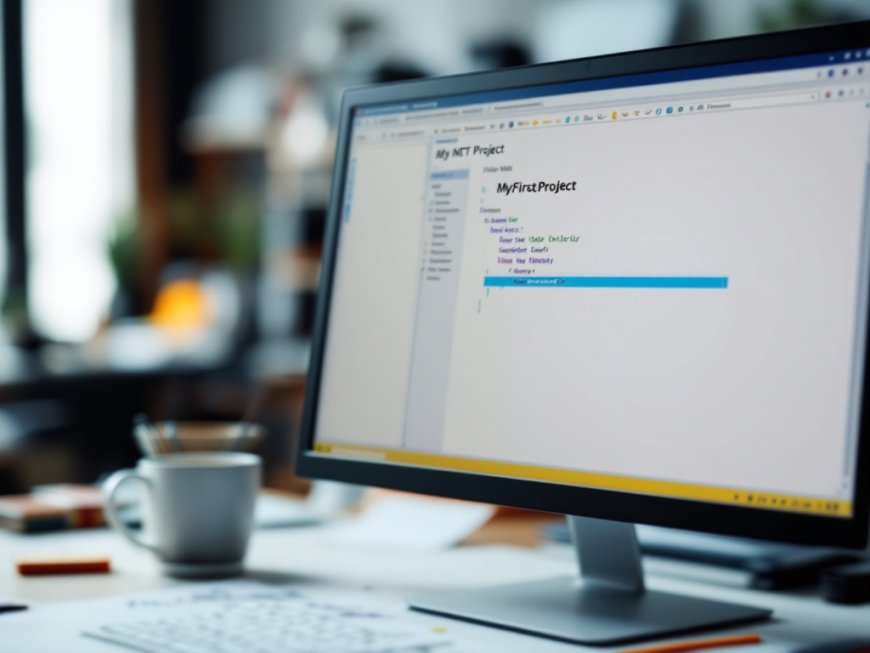
Are you ready to dive into the world of web development with Microsoft's powerful framework? Learning how to build your first project in ASP.NET can be an exciting journey that opens doors to creating dynamic, interactive web applications. This comprehensive guide will walk you through the entire process—from setting up your development environment to deploying your finished application. Whether you're a complete beginner or transitioning from another programming language, this article will provide you with the knowledge and confidence to get started.
Before diving deep into coding, it's helpful to understand what makes ASP.NET stand out. If you're considering a career in this field, a full stack .net course can give you the comprehensive skills needed to excel professionally. Additionally, familiarizing yourself with common dotnet core interview questions can prepare you for future job opportunities in this growing sector.
What is ASP.NET and Why Choose It?
ASP.NET is Microsoft's web development framework designed to build modern web applications and services. It's part of the larger .NET platform and allows developers to create web applications using languages like C#, VB.NET, and F#. Before you learn how to build your first project in ASP.NET, understanding its advantages is important:
-
Cross-platform compatibility: Modern ASP.NET Core works on Windows, macOS, and Linux
-
High performance: It's engineered for speed and efficiency
-
Robust security features: Built-in protection against common vulnerabilities
-
Extensive libraries: Access to a vast ecosystem of components and packages
-
Strong community support: A wealth of resources and active developer forums
Setting Up Your Development Environment
How to build your first project in ASP.NET begins with setting up the right tools. Here's what you'll need:
Installing Visual Studio
Visual Studio is the preferred integrated development environment (IDE) for ASP.NET development. Follow these steps:
-
Download Visual Studio from Microsoft's official website
-
Choose the Community Edition (free) or a paid version if you have a license
-
During installation, select the "ASP.NET and web development" workload
-
Complete the installation and launch Visual Studio
Installing .NET SDK
The .NET Software Development Kit (SDK) contains everything you need to build and run .NET applications:
-
Visit the official .NET download page
-
Download the latest stable version of the .NET SDK
-
Follow the installation instructions for your operating system
-
Verify the installation by opening a command prompt and typing dotnet --version
Creating Your First ASP.NET Project
Now that your environment is ready, let's focus on how to build your first project in ASP.NET:
Starting a New Project in Visual Studio
-
Open Visual Studio
-
Click on "Create a new project"
-
In the search box, type "ASP.NET" to filter templates
-
Select "ASP.NET Core Web Application" and click "Next"
-
Name your project (e.g., "MyFirstASPApp") and choose a location to save it
-
Click "Create"
-
In the next dialog, select "Web Application (Model-View-Controller)" for a full-featured application
-
Ensure "Authentication" is set to "No Authentication" for simplicity
-
Click "Create" to generate the project
Understanding the Project Structure
When Visual Studio creates your project, you'll see several folders and files:
-
Controllers: Contains classes that handle HTTP requests
-
Models: Holds data model classes that represent your application's data
-
Views: Contains the UI templates (HTML with Razor syntax)
-
wwwroot: Stores static files like CSS, JavaScript, and images
-
Program.cs: The entry point for your application
-
Startup.cs: Configures services and the app's request pipeline
Building Basic Functionality
With the project structure in place, let's develop some basic functionality:
Creating a Model
Models represent the data of your application. Let's create a simple "Product" model:
-
Right-click on the "Models" folder and select "Add > Class"
-
Name it "Product.cs" and click "Add"
-
Replace the code with:
csharp
namespace MyFirstASPApp.Models
{
public class Product
{
public int Id { get; set; }
public string Name { get; set; }
public decimal Price { get; set; }
public string Description { get; set; }
}
}
Adding a Controller
Controllers handle user requests and return responses:
-
Right-click on the "Controllers" folder and select "Add > Controller"
-
Choose "MVC Controller - Empty" and click "Add"
-
Name it "ProductsController" and click "Add"
-
Implement basic CRUD (Create, Read, Update, Delete) operations
csharp
using Microsoft.AspNetCore.Mvc;
using MyFirstASPApp.Models;
using System.Collections.Generic;
namespace MyFirstASPApp.Controllers
{
public class ProductsController : Controller
{
// Sample data (in a real app, this would come from a database)
private static List<Product> products = new List<Product>
{
new Product { Id = 1, Name = "Laptop", Price = 999.99m, Description = "High-performance laptop" },
new Product { Id = 2, Name = "Smartphone", Price = 499.99m, Description = "Latest smartphone model" }
};
// GET: Products
public IActionResult Index()
{
return View(products);
}
// GET: Products/Details/5
public IActionResult Details(int id)
{
var product = products.Find(p => p.Id == id);
if (product == null)
{
return NotFound();
}
return View(product);
}
}
}
Creating Views
Views display the UI to users. Let's create views for our products:
-
Create an "Index" view:
-
Right-click inside the Index method and select "Add View"
-
Keep the default name "Index" and click "Add"
-
Replace the generated code with:
html
@model IEnumerable<MyFirstASPApp.Models.Product>
@{
ViewData["Title"] = "Products";
}
<h1>Products List</h1>
<p>
<a asp-action="Create">Create New</a>
</p>
<table class="table">
<thead>
<tr>
<th>@Html.DisplayNameFor(model => model.Name)</th>
<th>@Html.DisplayNameFor(model => model.Price)</th>
<th>Actions</th>
</tr>
</thead>
<tbody>
@foreach (var item in Model)
{
<tr>
<td>@Html.DisplayFor(modelItem => item.Name)</td>
<td>@Html.DisplayFor(modelItem => item.Price)</td>
<td>
<a asp-action="Details" asp-route-id="@item.Id">Details</a>
</td>
</tr>
}
</tbody>
</table>
-
Create a "Details" view using similar steps
Working with Data
In real applications, you'll want to persist data in a database. Here's how you can integrate Entity Framework Core:
Adding Entity Framework Core
-
Install required NuGet packages:
-
Right-click on the project in Solution Explorer
-
Select "Manage NuGet Packages"
-
Search for and install:
-
Microsoft.EntityFrameworkCore
-
Microsoft.EntityFrameworkCore.SqlServer
-
Microsoft.EntityFrameworkCore.Tools
-
Create a database context class:
-
Add a new folder called "Data"
-
Add a new class called "ApplicationDbContext.cs"
-
Implement the context:
csharp
using Microsoft.EntityFrameworkCore;
using MyFirstASPApp.Models;
namespace MyFirstASPApp.Data
{
public class ApplicationDbContext : DbContext
{
public ApplicationDbContext(DbContextOptions<ApplicationDbContext> options)
: base(options)
{
}
public DbSet<Product> Products { get; set; }
}
}
-
Register the database context in Startup.cs:
csharp
public void ConfigureServices(IServiceCollection services)
{
services.AddDbContext<ApplicationDbContext>(options =>
options.UseSqlServer(Configuration.GetConnectionString("DefaultConnection")));
services.AddControllersWithViews();
}
-
Add a connection string in appsettings.json:
json
"ConnectionStrings": {
"DefaultConnection": "Server=(localdb)\\mssqllocaldb;Database=MyFirstASPApp;Trusted_Connection=True;MultipleActiveResultSets=true"
}
Creating and Applying Migrations
With Entity Framework Core, you can use migrations to create and update your database schema:
-
Open the Package Manager Console (Tools > NuGet Package Manager > Package Manager Console)
-
Create a migration: Add-Migration InitialCreate
-
Apply the migration to create the database: Update-Database
Enhancing Your Application
Now that we have the basics in place, let's enhance our application:
Adding Form Validation
ASP.NET provides easy-to-use validation features:
-
Update your Product model with validation attributes:
csharp
using System.ComponentModel.DataAnnotations;
namespace MyFirstASPApp.Models
{
public class Product
{
public int Id { get; set; }
[Required]
[StringLength(100)]
public string Name { get; set; }
[Required]
[Range(0.01, 10000)]
[DataType(DataType.Currency)]
public decimal Price { get; set; }
[StringLength(500)]
public string Description { get; set; }
}
}
-
Update controller actions to validate model state:
csharp
[HttpPost]
[ValidateAntiForgeryToken]
public async Task<IActionResult> Create(Product product)
{
if (ModelState.IsValid)
{
_context.Add(product);
await _context.SaveChangesAsync();
return RedirectToAction(nameof(Index));
}
return View(product);
}
Implementing Authentication and Authorization
For applications requiring user accounts:
-
Create a new project with Individual User Accounts authentication
-
Use the [Authorize] attribute to restrict access to certain controllers or actions
-
Implement role-based authorization for more fine-grained control
Styling Your Application
Enhance the visual appeal of your application:
-
Use Bootstrap (included by default) for responsive layouts
-
Customize the site.css file in the wwwroot/css folder
-
Consider using a modern CSS framework like Tailwind CSS
Testing and Debugging
Testing is crucial for building reliable applications. In ASP.NET, you can:
-
Create unit tests for your controllers and models
-
Use the built-in debugging tools in Visual Studio
-
Set breakpoints and inspect variables during execution
-
Use the Developer Exception Page during development
Deploying Your ASP.NET Application
Once your application is ready, you can deploy it to various environments:
Publishing to IIS
-
Right-click on your project and select "Publish"
-
Choose "IIS, FTP, etc." as the target
-
Configure the publish settings
-
Click "Publish" to deploy your application
Deploying to Azure
Microsoft Azure provides excellent hosting options for ASP.NET applications:
-
Right-click on your project and select "Publish"
-
Choose "Azure" as the target
-
Select "Azure App Service" and follow the wizard
-
Configure your Azure resources and deploy
Next Steps After Your First Project
After successfully building your first project in ASP.NET, consider these next steps:
-
Learn about ASP.NET Web API for building RESTful services
-
Explore Blazor for building interactive web UIs with C#
-
Dive deeper into Entity Framework Core for advanced data access
-
Study authentication and authorization in detail
-
Learn about continuous integration and deployment
How to build your first project in ASP.NET is just the beginning of your journey. As you continue to explore and build more complex applications, you'll discover the true power and flexibility of the ASP.NET framework. Remember that web development is a continuous learning process, and each project will help you grow as a developer.
Common Challenges and Solutions
As a beginner, you might encounter some challenges. Here are common issues and their solutions:
Runtime Errors
-
Use try-catch blocks to handle exceptions gracefully
-
Check the detailed error messages in the developer console
-
Review your application's logs for additional information
Database Connection Issues
-
Verify your connection string in appsettings.json
-
Ensure your database server is running
-
Check firewall settings that might block connections
Performance Problems
-
Use caching for frequently accessed data
-
Optimize database queries with proper indexing
-
Minimize the use of synchronous operations that block threads
Conclusion
Building your first project in ASP.NET is an important milestone in your development journey. By following this guide, you've learned the fundamentals of creating web applications using Microsoft's powerful framework. You've set up your development environment, created a basic application structure, implemented data access, added user interface components, and prepared your application for deployment.
Remember that mastering ASP.NET takes time and practice. Continue experimenting with different features, explore advanced topics, and build increasingly complex applications to enhance your skills. With dedication and persistence, you'll soon be creating robust, scalable web applications that solve real-world problems.
The web development landscape continually evolves, so stay updated with the latest ASP.NET features and best practices. Join developer communities, participate in forums, and collaborate with other developers to share knowledge and experiences. How to build your first project in ASP.NET is just the beginning—there's a whole world of web development waiting for you to explore.
Frequently Asked Questions (FAQs)
What programming languages can I use with ASP.NET?
ASP.NET supports multiple languages, but C# is the most commonly used. You can also use VB.NET or F#. C# is particularly popular due to its modern syntax, strong typing, and extensive community support.
Do I need prior programming experience to learn ASP.NET?
While prior programming experience is helpful, it's not absolutely necessary. However, understanding basic programming concepts like variables, loops, and conditionals will make learning ASP.NET much easier. Familiarity with HTML and CSS is also beneficial.
Is ASP.NET free to use?
Yes, ASP.NET is free to use. You can download and use Visual Studio Community Edition, the .NET SDK, and all the core libraries at no cost. This makes it accessible for hobbyists, students, and small businesses.
What's the difference between ASP.NET and ASP.NET Core?
ASP.NET Core is the newer, cross-platform, high-performance version of ASP.NET. While traditional ASP.NET runs only on Windows, ASP.NET Core works on Windows, macOS, and Linux. ASP.NET Core is also more modular and has better performance.
Can I create mobile applications with ASP.NET?
ASP.NET itself is for web applications, but you can create responsive websites that work well on mobile devices. For native mobile apps, you might want to look at Xamarin (now part of .NET MAUI), which allows you to use C# for cross-platform mobile development.
What database systems work with ASP.NET?
ASP.NET works with virtually any database system. SQL Server is the most common choice due to Microsoft integration, but you can also use MySQL, PostgreSQL, SQLite, MongoDB, and many others through various providers.
How do I handle user authentication in ASP.NET?
ASP.NET offers built-in authentication systems. You can use ASP.NET Identity for traditional username/password authentication, or integrate with external providers like Microsoft, Google, Facebook, etc. For more advanced scenarios, you might use IdentityServer or Auth0.
Is ASP.NET suitable for large-scale applications?
Absolutely. ASP.NET is designed to be scalable and is used by many large enterprises for mission-critical applications. Its performance, security features, and support for enterprise patterns make it suitable for applications of any size.
How can I deploy my ASP.NET application for free?
Several platforms offer free tiers for ASP.NET applications, including Azure App Service (with limitations), Heroku, and Netlify. For development and testing, you can use IIS Express or Kestrel locally at no cost.
What resources are available for learning more about ASP.NET?
Microsoft provides excellent documentation at docs.microsoft.com. Other valuable resources include Pluralsight courses, YouTube tutorials, GitHub samples, Stack Overflow, and various blogs from ASP.NET community members. Microsoft's official samples repository is also a great place to learn by example.